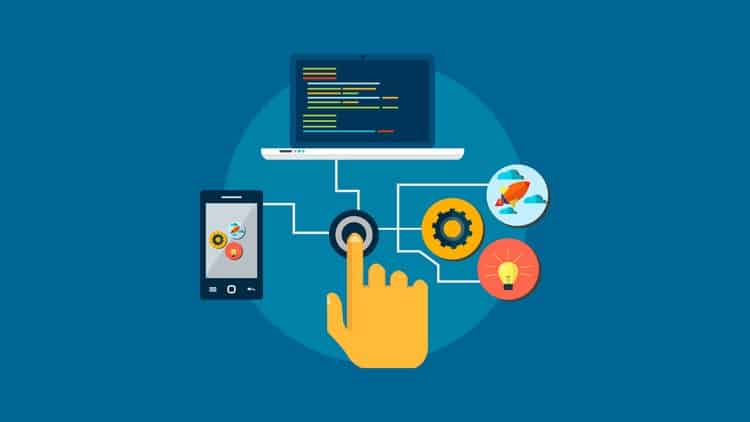
Most websites and web based applications interact with a database in many ways, either to provide or store information. For example, if you think about a website with a members area, it has a registration and login form. When you register for an account the information is sent and stored in a table within a database. When you login to the website it queries your login information with what’s in the database. Thats just a basic example, but databases are heavily used all the time for many different things.
This blog for example uses a database to store various information, like the blog posts, comments, tags, image paths, user accounts and so on.
In this example we are going to look at how to insert data into a sql table using php and mysqli.
Here’s the code to insert data
$activity = mysqli_query($connect, “INSERT INTO meds
(med_name, med_type, med_mg, addedby, dateadded) VALUES (‘$medname’, ‘$medtype’, ‘$medmg’, ‘$addedby’, ‘$ddate’)”);
All the variables and column names are related to a project, you will need to change them based on your own needs.
Most data that gets added normally inosines a user input, so the below is an example of a form that then submits the data to a table.
Example of a html form used to insert data
<?php if(isset($_POST['addmed'])){
$medname = $_POST['medname'];
$medname = mysqli_real_escape_string($connect, "$medname");
$medtype = $_POST['medtype'];
$medtype = mysqli_real_escape_string($connect, "$medtype");
$medmg = $_POST['medmg'];
$medmg = mysqli_real_escape_string($connect, "$medmg");
$addedby = $_POST['addedby'];
$addedby = mysqli_real_escape_string($connect, "$addedby");
echo"Medication added <BR><BR>";
$ddate = date("Y-m-d H:i:s");
$activity = mysqli_query($connect, "INSERT INTO `meds` (med_name, med_type, med_mg, addedby, dateadded) VALUES ('$medname', '$medtype', '$medmg', '$addedby', '$ddate')");
}
?>
<form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>">
<input type="text" name="medname" placeholder="Medication name" required><br>
<select class="select-css" name="medtype" required>
<option value="Tablet">Tablet</option> <br>
<option value="Liquid">Liquid</option> <br>
<option value="Injection">Injection</option> <br>
</select> Type <br>
<input type="number" name="medmg" placeholder="0" required> mg<br>
<input type="text" name="addedby" placeholder="<?=$uname?>" readonly><br>
<input type="submit" name="addmed" value="Add Medication">
</form>
Feel free to copy and paste the code, you will need to modify it to suit your own project and structure.